Toggle Switch
View in LexiconToggle provide users with different selection and activation tools.
install | yarn add @clayui/form |
---|---|
version | |
use | import {ClayToggle} from '@clayui/form'; |
Table of Contents
Example
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle"></span>
</span>
</span>
</label>
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span
className="toggle-switch-handle"
data-label-off=""
data-label-on="LIVE"
>
<span className="button-icon button-icon-on toggle-switch-icon">
<svg
className="lexicon-icon lexicon-icon-live"
focusable="false"
role="presentation"
>
<use href="/images/icons/icons.svg#live"></use>
</svg>
</span>
<span
className="button-icon button-icon-off toggle-switch-icon"
>
<svg
className="lexicon-icon lexicon-icon-staging"
focusable="false"
role="presentation"
>
<use href="/images/icons/icons.svg#staging"></use>
</svg>
</span>
</span>
</span>
</span>
</label>
Behavioral
You may want the Toggle Switch to behave with a Radio or Checkbox but have the appearance of a switch.
Checkbox
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle"></span>
</span>
</span>
</label>
Radio
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input
className="toggle-switch-check"
name="toggleSwitchRadio1"
role="radio"
type="radio"
value="option1"
/>
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle"></span>
</span>
</span>
</label>
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input
className="toggle-switch-check"
name="toggleSwitchRadio1"
role="radio"
type="radio"
value="option2"
/>
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle"></span>
</span>
</span>
</label>
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input
className="toggle-switch-check"
name="toggleSwitchRadio1"
role="radio"
type="radio"
value="option3"
/>
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle"></span>
</span>
</span>
</label>
Disabled
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input
disabled
class="toggle-switch-check"
role="switch"
type="checkbox"
/>
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle"></span>
</span>
</span>
</label>
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input
disabled
checked
className="toggle-switch-check"
name="toggleSwitchRadio1"
role="radio"
type="radio"
value="option1"
/>
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle"></span>
</span>
</span>
</label>
Composable
With Text
You can display additional text with the toggle switch by adding the .toggle-switch-text
class to the text element. Use the .toggle-switch-text-left
and .toggle-switch-text-right
classes to position the text on the left and right side of the toggle switch, respectively.
<label className="toggle-switch">
<span className="toggle-switch-label">Adding Required Text</span>
<span className="toggle-switch-text">Required *</span>
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span
className="toggle-switch-handle"
data-label-off=""
data-label-on="ON"
>
</span>
</span>
</span>
</label>
<label className="toggle-switch">
<span className="toggle-switch-label">Adding Required Text</span>
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span
className="toggle-switch-handle"
data-label-off=""
data-label-on="ON"
>
</span>
</span>
</span>
<span className="toggle-switch-text">Required *</span>
</label>
<label className="toggle-switch">
<span className="toggle-switch-label">Required Text on Right</span>
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span
className="toggle-switch-handle"
data-label-off=""
data-label-on="ON"
>
</span>
</span>
</span>
<span className="toggle-switch-text toggle-switch-text-right"
>Required *</span
>
</label>
<label className="toggle-switch">
<span className="toggle-switch-label">Required Text on Left</span>
<span className="toggle-switch-text toggle-switch-text-left"
>Required *</span
>
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span
className="toggle-switch-handle"
data-label-off=""
data-label-on="ON"
>
</span>
</span>
</span>
</label>
<label className="toggle-switch">
<span className="toggle-switch-label">The Kitchen Sink</span>
<span className="toggle-switch-text">Top Text</span>
<span className="toggle-switch-text toggle-switch-text-left">Error</span>
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span
className="toggle-switch-handle"
data-label-off="OFF"
data-label-on="ON"
>
</span>
</span>
</span>
<span className="toggle-switch-text toggle-switch-text-right"
>Required *</span
>
<span className="toggle-switch-text">Bottom Text</span>
</label>
With Icons
Add <span className="button-icon button-icon-on icon-volume-up toggle-switch-icon"/>
to add an icon to the switch for the on position.
Add <span className="button-icon button-icon-off icon-volume-off toggle-switch-icon"/>
to add an icon to the switch for the off position.
Alternatively, you can add <span className="icon-ok toggle-switch-icon toggle-switch-icon-on"/>
to add an icon to the switch for the on position.
Alternatively, you can add <span className="icon-remove toggle-switch-icon toggle-switch-icon-off"/>
to add an icon to the switch for the off position.
<label className="toggle-switch">
<span className="toggle-switch-check-bar">
<input className="toggle-switch-check" role="switch" type="checkbox" />
<span aria-hidden="true" className="toggle-switch-bar">
<span className="toggle-switch-handle">
<span className="toggle-switch-icon toggle-switch-icon-on">
<svg
className="lexicon-icon lexicon-icon-check"
focusable="false"
role="presentation"
>
<use href="/images/icons/icons.svg#check" />
</svg>
</span>
<span className="toggle-switch-icon toggle-switch-icon-off">
<svg
className="lexicon-icon lexicon-icon-times"
focusable="false"
role="presentation"
>
<use href="/images/icons/icons.svg#times" />
</svg>
</span>
</span>
</span>
</span>
</label>
Extending Toggles
This section explains how to customize toggles. Use at your own risk.
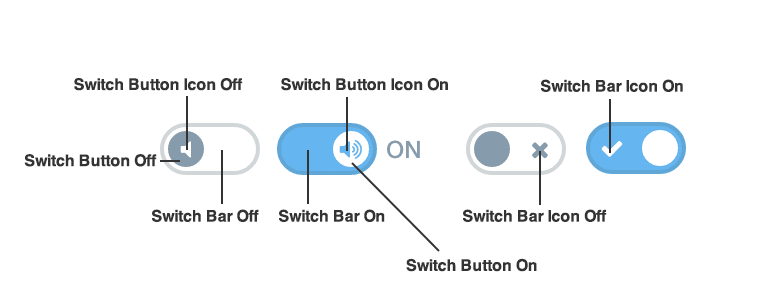
-
Customize the
toggle-switch button in the off
position with
.toggle-switch-check:empty ~ .toggle-switch-bar:after {}
. -
Customize the
toggle-switch button icon in the off
position with
.toggle-switch-check:empty ~ .toggle-switch-bar .toggle-switch-icon.button-icon {}
. -
Customize the
toggle-switch bar in the off
position with
.toggle-switch-check:empty ~ .toggle-switch-bar:before {}
. -
Customize the
toggle-switch bar icon in the off
position with
.toggle-switch-check:empty ~ .toggle-switch-bar .toggle-switch-icon-off {}
. -
Customize the
toggle-switch button in the on
position with
.toggle-switch-check:checked ~ .toggle-switch-bar:after {}
. -
Customize the
toggle-switch button icon in the on
position with
.toggle-switch-check:checked ~ .toggle-switch-bar .toggle-switch-icon.button-icon.
-
Customize the
toggle-switch bar in the on
position with
.toggle-switch-check:checked ~ .toggle-switch-bar:before {}
. -
Customize the
toggle-switch bar icon in the on
position with
.toggle-switch-check:checked ~ .toggle-switch-bar .toggle-switch-icon-on {}
.